Must-have toolchain for full stack development
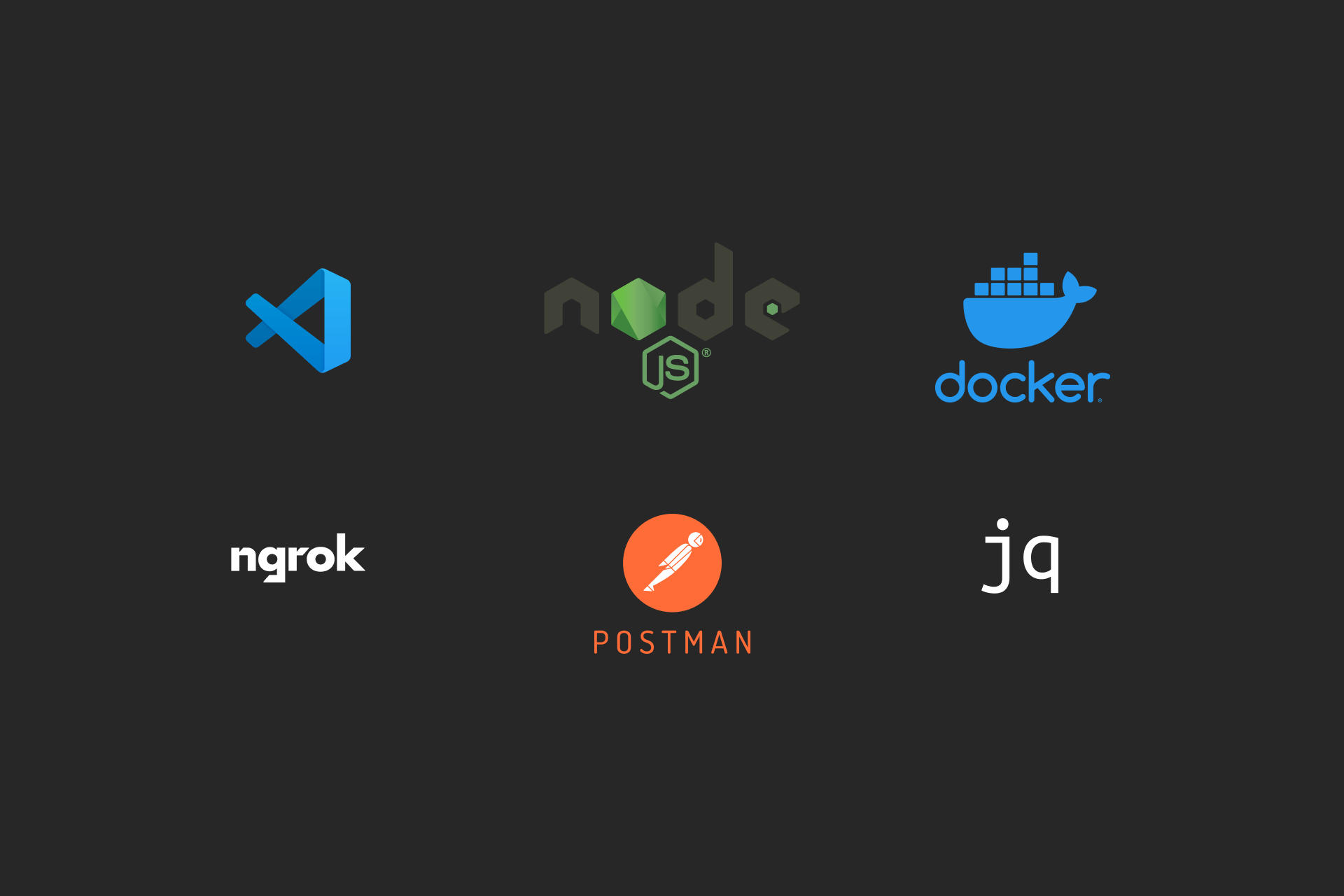
Here are the essential tools I think you need to get started on your full-stack development journey with JavaScript. They are tools that are either strictly required, or at minimum be greatly helpful in your development journey. In most of the things I’ve included installation links or instructions for linux and MacOS, but of course in theory you could also do it all on Windows. But please, don’t.
NodeJS
NodeJS is the engine responsible for running JavaScript outside of the browser. It is a must for developing modern websites and backends written in JavaScript. NodeJS can run almost any JavaScript code directly from a .js
file, with the exception of not having access to the window
object, as obviously there is no browser window to access.
node hello-world.js
To install NodeJS on Linux or MacOS I would highly recommend using NVM, Node Version Manager, which allows you to conveniently have multiple versions of Node installed and makes installing a new version a breeze. To install it, use the following script:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
Then installing a specific version of NodeJS is as simple as:
# Install NodeJS v16
nvm install 16
# Use v16 in the current shell
nvm use 16
# Set v16 as the default for new shells
nvm alias default 16
NodeJS comes with NPM, the Node Package Manager, which manages dependency libraries for your project. One popular alternative for NPM is Yarn, which was built by Facebook to address issues in the early versions of NPM. Nowadays though they are very similar in capabilities and whichever you want to use is up to you. To learn more about NPM and Yarn, check out this great article on ImaginaryCloud.
Starting new projects with NPM is super easy, just use the npm init
command. By default, it asks you questions to fill in fields in the resulting package.json
. Most of the time, however, the default values are fine and you can use them by repeatedly pressing enter or instead give the --yes
option directly to the init
command. So, to start a new project:
mkdir my-new-project && cd my-new-project
npm init --yes
npm install typescript
VSCode & extensions
My favourite code editor is definitely VSCode by Microsoft. It’s fast, beautiful, powerful and easily extensible to suit your needs. And best of all it’s completely free and built on open source code. There is a million extensions for anything from generating random strings and numbers to automatically closing html tags.
If you’re using it on MacOS, I would recommend adding an alias to your ~/.bash_profile
(or wherever you have your aliases) to make it easy to open any file or folder directly from your terminal with code <file or folder>
. If you’ve installed it on linux, it should have already come preinstalled with this shortcut.
alias code='open -a "/Applications/Visual Studio Code.app"'
Here are my favourite extensions that I could not code anymore without:
- Auto Close Tag - Automatically add closing HTML/XML tag when you write an opening one.
- Auto Rename Tag - Automatically rename closing HTML/XML tag when renaming the opening one. This is very useful when refactoring React code.
- Rainbow Brackets - Colorizes paired parentheses and brackets with rainbow colors, making it easier to see matching brackets and their depth.
- vscode-styled-components - Adds CSS syntax highlighting and autocomplete for CSS in JS code.
- Random Everything - Generate random strings, numbers, emails, names etc. for your dummy data.
Docker
Docker is the magic tool that allows you to run almost any software you want without installing anything except Docker on your computer. It lets you be 100% sure that if it runs on your machine, it will run on any machine (with Docker installed). And it makes deploying your software on a new server happen in seconds, not in minutes or hours.
In practice, you package your software and all its dependencies (build
) inside a Docker Container. Then you can transfer that container (push
) to a registry to be deployed (pull
) from to wherever you want to run it at. You won’t need to worry about installation or dependencies, you can simply just run
it.
To install Docker on MacOS, download and install Docker Desktop. On linux, you can either install just the Docker Engine without the desktop client, or the desktop version similar to MacOS. Personally I never use the desktop client for anything and on MacOS it’s really just an annoyance, but unfortunately on Mac it’s the only way to install Docker. No matter which version you get, you’ll then have access to the docker
command in your shell.
docker run -it busybox echo "Hello world!"
Docker Container is similar to a virtual machine, but it does not emulate the computer hardware. That makes it much smaller, lighter and faster to run than a virtual machine. The only caveat is that a docker container must be built for the same computer architecture as where you will be running it. That means that if you’re using a new ARM based M1 or M2 Mac to build your docker containers, and are planning to run those containers on an amd64 based system (e.g. in the cloud), just remember to add the target platform to the build command or running your container will fail with very little info on why.
docker build --platform linux/amd64 -t my-container .
If you want to get more familiar with Docker, checkout this great (albeit long) tutorial on all things Docker. Or, if you’re short on time, this quickstart on just the basic commands. Then when you’re familiar with it and are feeling adventurous, you can try running VSCode in the cloud in a docker container!
Postman or Insomnia
Both Postman and Insomnia are practically just a GUI for curl. You can easily send HTTP requests and see the responses automatically pretty printed. That’s cool and all, but what makes them an invaluable tool in your full-stack toolbox is saving and organizing the requests to serve as both documentation and easy access to the API you are working on or with. You can create environments to send the same requests easily to different servers (e.g. local, staging and production), asserts to check that the responses are as you expect, and run a whole bunch of requests together to serve as a full API test suite.
Collections in Postman or Insomnia are a great way to record all your backend endpoints, by saving an example request complete with parameters, headers and request body. You can export the collection into a file and save it together with your code. That way you’ll never have to guess how to call in that backend you worked on a year ago, but instead you’ll have the request already prepared and ready to send.
Personally I’ve used Postman for a long long time already, but there is no particular reason other than habit why I’d choose Postman over Insomnia. Only thing is Postman has recently become a bit pushy with their sign up and paid versions, so maybe one day I will get bored and change to Insomnia. In any case, they both work great, so find your own preference!
ngrok
Whenever you’re developing frontends or backends, there often comes a time when you would like to have your localhost server accessible from the internet. Maybe you would like to test receiving webhook events, have other online services call your local server or just want to show off your work to your friends. With ngrok, that is as easy as a one-liner in your terminal! It gives you a public URL and forwards all requests received to that URL to your local server.
ngrok http 3000
This little command creates a URL and forwards all HTTP traffic to the server running in your localhost port 3000. That’s easy, right? And best of all, it’s completely free! You’ll only need to sign up for a free account to ngrok.
jq
When working with APIs (either using or creating), you’ll inevitably have to deal with JSON data. jq
is a command line tool that in its simplest form can pretty print JSON strings in your terminal.
echo '{"hello":"world"}' | jq
This is handy for printing API responses in a more understandable fashion:
curl -s https://api.sampleapis.com/coffee/hot | jq
But, of course, it can do A LOT more. You can use it to manipulate JSON documents in any which way, e.g. filtering, combining or transforming them. The easiest function is select, returning only the wanted fields in documents. For example, to only get a list of IDs for the hot coffee beverages, you would do:
curl -s https://api.sampleapis.com/coffee/hot | jq '.[].id'
jq
is a very powerful tool when working with JSON data, and it can save you a big bunch of time in developing or debugging things. You can also use it in scripts, passing select data from one API’s response to another API call. The possibilities are endless!
To install jq
on MacOS you can use Homebrew, and on linux you can install it with your favourite package manager:
brew install jq
# OR
sudo apt-get install jq
Conclusion
Those are the 6 must-have tools I think every full-stack developer should have to get started. The internet is full of great and not-so-great tools and software, but these are the ones I really could not do without. Can you think of something else that is absolutely essential? Please let me and your fellow devs know down in the comments!